Using Sliders and Lift Curves in Unity
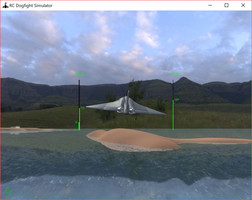
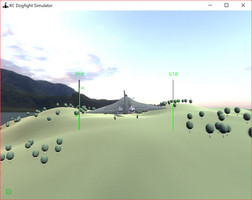
After so long seeing text on the side of the screen, an actual HUD is a relief to me! Implementing it was not too difficult after all.
For example, adding throttle power was just a matter of adding a slider to the HUD's canvas:
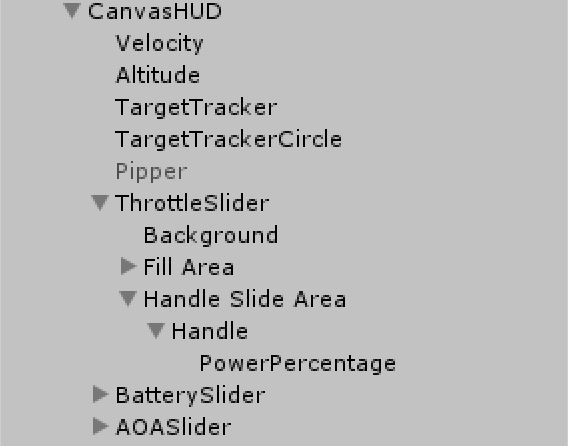
The power percent text that moves along with the green bar is made a child of the handle (and the handle is given 0 height to make it disappear). Then, to make the slider reflect the current throttle setting, in code, this is written as part of the HUD's script:
m_ThrottleSlider.value = throttle;
Because the slider's minimum-maximum value is set in the inspector as 0-1, if the throttle is at 50% (or 0.5), the slider will be set at middle -- and so on. The rest is just fiddling with the slider's colour and size.
To display the correct throttle percent numerically, we write:
m_ThrottlePercentage.text = ((int)(100 * throttle)).ToString();
Where m_ThrottlePercentage is a Text variable referencing the PowerPercentage game object in the inspector. By multiplying throttle by 100, we turn a percent (eg. 0.5) into an floating point representation (eg. 50.0), then typecast that number using (int) to return only the integer value (eg. 50).
Implementing Lift Curves
In this version, lift has been changed as well. In the past, lift depended on a single value and varied based on angle of attack. However, this does not take into account various wing shapes (straight, swept, and delta). Therefore, I changed my flight script and added an animation curve representing lift/angle of attack:
Based on the following variable:
[SerializeField] public AnimationCurve m_WingLift; // A picture of lift to angle of attack
Now, how will angle of attack be accounted for? Remember that an animation curve goes over time, generally from 0-1 seconds. So by using angle as a percent, we can know where the wing is over the curve, representing how much lift the wing generates. This is done using a C# property named Lift:
public float Lift { get { float lift = m_WingLift.Evaluate(Mathf.Clamp01(Mathf.Abs(ForceOfAttack))); return Mathf.Clamp01(lift); } }
The takeaway here is AnimationCurve.Evaluate(), or, in this case, m_WingLift.Evaluate(Mathf.Clamp01(Mathf.Abs(ForceOfAttack))), which uses the absolute value of ForceOfAttack, clamped from 0-1, to return a value along the lift curve as a percent of ForceOfAttack. This is a little messy for now, but basically means that the more the airplane's wings are forcing against the wind, according to the ForceOfAttack variable, the more the value returned will be towards the right on the animation curve.
To be more accurate, this calculation could include another curve for drag, which increases exponentially with angle of attack; but because of time and technicalities, I didn't sort that out yet. My flight model is not perfect, but I am excited to be able to simulate custom wings (eg. glider wings vs. delta wings).
To apply the lift, we do the following:
// Accumulate forces acting onto the airplane Vector3 forces = Vector3.zero; // Add engine power forward forces += EnginePower * transform.forward; // Apply lift at right angles to the airplane's velocity (usually 'up') Vector3 liftDirection = Vector3.Cross(m_Rigidbody.velocity, transform.right).normalized; // Calculate and add the lift power; AoA changes add drag, but also add lift when pointing up float liftPower = ForwardSpeed * Lift * m_WingLoadFactor; forces += (liftPower - m_Rigidbody.drag) * liftDirection; // Apply the calculated forces to the the Rigidbody m_Rigidbody.AddForce(forces);
In the above code, the following line:
float liftPower = ForwardSpeed * Lift * m_WingLoadFactor;
Applies lift according to our Lift property previously defined. m_WingLoadFactor is just a percent that tells the program how much we want lift to affect the airplane. This is basically a way of telling our program how large our wings are in relation to the airplane's body (eg. a glider vs. a jet).
Hope you enjoyed the process of adding curves and sliders in Unity as much as I am enjoying refining my flight model and appearance!
Files
Get Remote Control Dogfight Simulator
Remote Control Dogfight Simulator
Battle EDF jets using first-person cameras, lasers detectors, and compressed-air missiles
Status | Prototype |
Author | Denis Labrecque |
Genre | Simulation |
Tags | airplane, laser, radio, remote-control |
Languages | English |
More posts
- First Network-Playable Version!Sep 13, 2018
Leave a comment
Log in with itch.io to leave a comment.